Motor
Unipolar stepper motor

Bipolar Stepper Motor
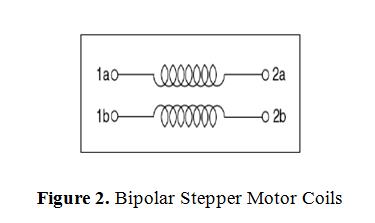
Variable Reluctance stepper motor (hybrid stepper motors)

Driving Unipolar Stepper Motors



Two Phases ON Mode (Alternate Full step Mode)



Half Step Mode




Application:
1. They are relevant in consumer office equipment such as printers, plotters, copiers, and scanners.
/* Name : main.c * Purpose : Source code for interface Stepper Motor with 8051 Microcontroller (AT89C52) * Author : vijayaraja R * Date : 2014-01-22 * Website : www.gemicates.org * Revision : None */ /**** Full step drive Mode****/ #include <REGX52.H> #include<stdio.h> #define motor P2 void delay(int sec); void main() //main function { do { motor = 0x03; //0011 delay(100); motor = 0x06; //0110 delay(100); motor = 0x0C; //1100 delay(100); motor = 0x09; //1001 delay(100); } while(1); } void delay(int sec) //Function provide msec delay { int i,j; for(i=0;i<sec;i++) for(j=0;j<1000;j++); }
/* Name : main.c * Purpose : Source code for interface Stepper Motor with 8051 Microcontroller (AT89C52) * Author : vijayaraja R * Date : 2014-01-22 * Website : www.gemicates.org * Revision : None */ /**** Half Drive Stepping Mode ****/ #include <REGX52.H> sfr stepper=0xA0; // GPIO P2 declaration void delay(unsigned int count) { int i,j; for(i=0;i<count;i++) for(j=0;j<1025;j++); } void main() // main function { while(1) { stepper=0x01; delay(100); stepper=0x03; delay(100); stepper=0x02; delay(100); stepper=0x06; delay(100); stepper=0x04; delay(100); stepper=0x0C; delay(100); stepper=0x08; delay(100); stepper=0x09; delay(100); } }
/* Name : main.c * Purpose : Source code for interface Stepper Motor with 8051 Microcontroller (AT89C52) * Author : vijayaraja R * Date : 2014-01-17 * Website : www.gemicates.org * Revision : None */ /**** Wave Drive Stepping Mode****/ /******Single-Coil Excitation******/ #include <REGX52.H> #include<stdio.h> #define motor P2 void delay(int sec); void main() // main function { do { motor=0x01; // 0001 delay(1000); motor=0x02; // 0010 delay(1000); motor=0x04; // 0100 delay(1000); motor=0x08; // 1000 delay(1000); } while(1); } void delay(int sec) // Function provide msec delay { int i,j; for(i=0;i<sec;i++) { for(j=0;j<100;j++) {} } }
/* Name : main.c * Purpose : Source code for interface Bipolar Stepper Motor with 8051(AT89C52). * Author : Gemicates * Date : 2014-01-22 * Website : www.gemicates.org * Revision : None */ /**** Bipolar Stepper Motor ****/ #include <REGX52.H> sfr stepper=0xA0; // GPIO P2 declaration void delay(int); void main() { do { stepper=0x01; //0001 delay(1000); stepper=0x04; //0100 delay(1000); stepper=0x02; //0010 delay(1000); stepper=0x08; //1000 delay(1000); }while(1); } void delay(int sec) { int i,j; for(i=0;i<sec;i++) { for(j=0;j<100;j++) {} } }